Selecting Elements
The $
Function
The main function in jQuery is the $
function. Yes, it's a dollar sign, but it's the basis of using jQuery. To select in element, we need to use the $
function. As an example, type the following code into script.js
:
$(document).ready(function(){
$("p").click(function(){
$(this).hide();
});
});
And then, in your index.html
file, add a couple of paragraph (p
) tags with text in them.
<p>Click Me!</p>
<p>Click Me Too!</p>
<p>No, Click Me!</p>
Code Result
After running your code, you will find a simple HTML page. Don't worry about the CSS, it doesn't matter. Well, the page looks bare, but if you click on one of the lines of text (ignore the cursor shape), then it disappears!
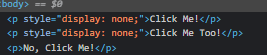
Code Breakdown
Now, let's see how the $
function works!
On the first line of the JavaScript code, we have
$(document).ready(function(){
which tells our web browser to ONLY run the code once the document, or web page, has loaded. Without it, our JavaScript code might try to access some elements that haven't been created yet on the page!!
Then, on the next line, we have
$("p").click(function(){
which tells jQuery to run the next couple fo lines of JavaScript ONLY when you click on a paragraph tag. In jQuery, the $ function takes strings or document elements (like the document object) and runs methods on those objects. The strings we pass in are the equivalent of CSS selectors.
Finally, other than the closing parantheses and brackets, we have
$(this).hide();
which tells jQuery: Ok, if someone clicks on one of the <p>
tags, then hide that p tag, but don't actually delete it. So, if we inspect the page after, we will see that jQuery added a CSS style for that p
element with display
set to none, so we can't see it and it won't affect with the flow of the page.
This is the basis of using jQuery selectors, but we'll learn a bit more about selecting elements in the next tutorial!