Your First Pygame Game
Writing the Code
The code will be as follows:
import pygame pygame.init() WIDTH, HEIGHT = 500, 500 WIN = pygame.display.set_mode((WIDTH, HEIGHT)) pygame.display.set_caption("Hello World!") run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False break pygame.quit()
Running the Code
To run the code, simply run it like you do a regular Python file.
Do not name your filepygame.py
as you import the Pygame module so naming your file pygame.py
will make it import itself. Nonsense!!
Code Breakdown
Now, let's break down the code!
First of all, we need to import Pygame using
import pygame
Without it, we wouldn't be able to use Pygame. If you get an error, however, that Pygame isn't defined, then make sure that you correctly setup Pygame.
On the next line, we have
pygame.init()which initializes Pygame. Without it, we won't be able to use Pygame for our games. It is often a good idea to write this line of code at the front of your code after all of your imports.
Then, we set up the screen dimensions in the variables WIDTH
and HEIGHT
. We do this so we can easily access the screen dimensions without needing to run any other functions.
On the next two lines, we have:
WIN = pygame.display.set_mode((WIDTH, HEIGHT)) pygame.display.set_caption("Hello World!")
We do this to setup the screen using pygame.display.set_mode
and then passing in the screen dimensions in the tuple (WIDTH, HEIGHT)
. Then, we set the caption, our the title, of our window. The default caption is pygame window
but we changed it to be Hello World!
which better suits are demo window.
And, finally, we have what we call an "Event Loop
" which every game will have. It is the loop that runs repeatedly and we put the code we want to run every single millisecond in their such as collision detection, drawing on the screen, etc.
Notice that, in our loop, we have a for loop that checks events. Basically, we use that code to check if the user presses/closes the "X" button or closes the game window and if they do, then we break out of the loop and close the window.
Code Result
After running the code, we get an empty black window, as seen below!
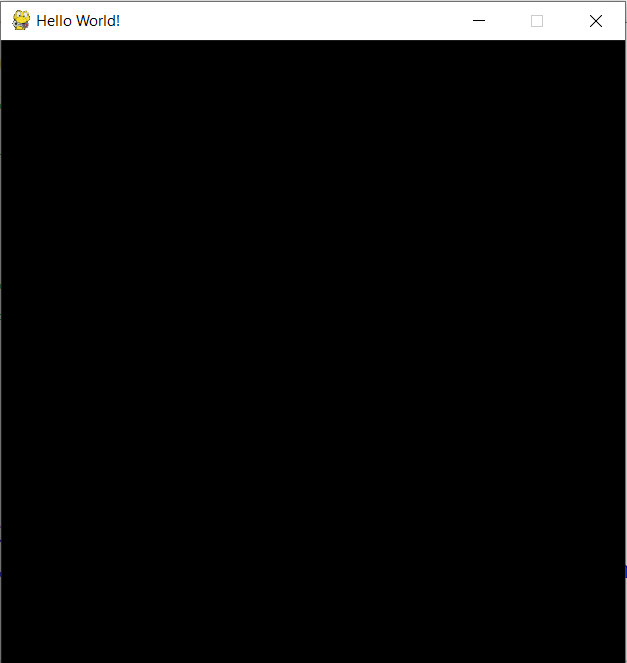
We will add more to that window in the next tutorial!