JavaScript Alerts
What is an Alert?
In JavaScript, an alert is a little message box on the webpage. Alerts are great for testing JavaScript code since you do need to go to the console, but alerts should generally not be overused because the web page cannot be used until the alert is closed.
Making an Alert
To make alerts, we use the alert()
function. You can run it from the console or a JavaScript file. For example, type the following code:
alert("Hello World!")
You should see something like:
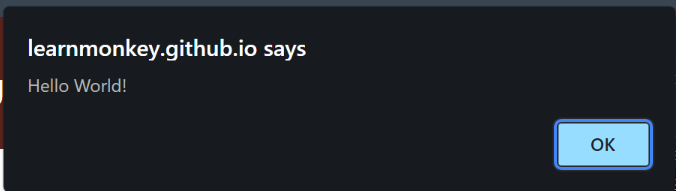
You can also view the demo alert by pressing the button:
Making a Confirmation Alert
In JavaScript, we also have the confirm()
function. confirm()
creates an alert box but with two buttons: OK and Cancel. If the user clicks OK, the function returns true
. If the user clicks Cancel, the function returns false
.
As an example, type the following code:
console.log(confirm("Are you sure you want to do this?"))
When you run it, you should see something like this:
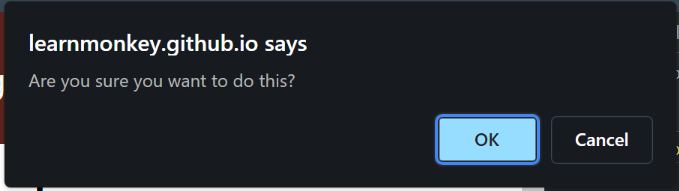
Click "OK". Now, go into the console. You should see true
.
If you do it again and click "Cancel", you should now see false
.
However, just like normal alerts, you should not overuse confirmation alerts. They do not allow the user to interact with the page until they click "OK" or "Cancel".
Making a Prompt Alert
The final type of alert that JavaScript offers in the prompt alert, which uses the prompt()
function. It allows the user to enter something in the alert. For example, write the following code:
console.log(prompt("Enter your favorite website:"))
When you run it, you should see something like this:
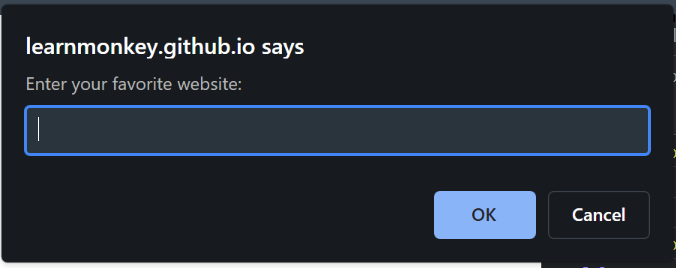
Then, you can enter "Learnmonkey" and click OK. Now, when you look at the console, you should see Learnmonkey
.
Now, do the same thing again but click "Cancel" instead. Then, you should see null
. null
basically means an empty value or variable.