Python Functions
What is a Function?
A function is similar to a variable, but instead of storing numbers or text, it stores lines of code for Python to run. Just like we learned in math class, functions in Python can have inputs.
Making Functions
To make a function, we use def
, which stands for "define". After that, we type our function name, which in this case is helloWorld
and type a pair of parentheses (()
). After that, we type a colon and press enter. Then, Sublime Text should indent the next line. This is because Python uses indentation for seperating blocks of code and this includes functions.
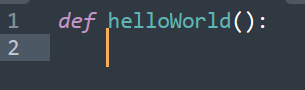
Then, inside of our function we can type
print("Hello World!")
. This tells Python that whenever we run the helloWorld
function, we want to print Hello World!.
Function Arguments and Parameters
When we are making our function, we can make our function have specific inputs so that when we use our function. These are called arguments. Then, when we run our functions, we can pass in the arguments we just defined as parameters. We can do it as follows:
def helloWorld(argument1, argument2, argument3, .....):
# do something
Running a function quite similar to making a function, but we don't use def
and we don't type anything after the parentheses. For example, we could call the helloWorld
fucntion we made earlier by typing helloWorld()
. When we run it, we get Hello World!.
Returning
When we are programming, we often want our functions to return something. We can do this in Python by using the return
keyword. In our function code, we simply type return [value]
, which will return the value. As an example, let's modify our helloWorld
function to return "Hello World!" instead of printing it. To do this, we type the following:
def helloWorld():
return "Hello World!"
Then, we can print the result of the function:
print(helloWorld())
As expected, we get back Hello World!.
Default Parameters
We can set default parameters as following:
def greet(name="Learnmonkey"):
print("Hello " + name)
greet()
The output of the code is Hello Learnmonkey.
Arbitrary Arguments (*args
)
In Python, we can have as many arguments as we want by putting *args
as one of the arguments, where args
is a tuple with all of the arguments. Below is an example:
*args
by typing args
not *args
.
def argsTest(*args):
print(args)
argsTest()
The output of the code is (1, 2.1, 3).
Note: We could rename*args
to *nums
or anything else. The only thing that matters in the asterisk in front. It is customary to use *args
.
Named Arguments
In Python, we can use named arguments to make our code more readable. When we are using named arguments, instead of putting out parameters in order, we can put our parameters in whatever order we choose. Below is an example of named arguments:
def favoriteFoods(food1, food2, food3):
print("My favorite foods are: ")
print(food1)
print(food2)
print(food3)
favoriteFoods(food2="noodles", food1="bananas", food3="pizza")
The output is:
bananas noodles pizza
As we can see, the order in which the parameters were placed doesn't matter.
Arbitrary Keyword Arguments (**kwargs
)
In Python, we have another similar thing to *args
which is **kwargs
. It's similar in the sense that they start with asterisks, only that **kwargs
has two asterisks but with **kwargs
, but **kwargs
takes arguments as seperate parameters. For example, if I have the code
def my_function(**stuff):
print(stuff)
my_function(random=2765, thing="gega", my_name="monkey")
Fun fact: the kw
in kwargs
stands for keyword arguments.
After running that code, we get a dictionary, where the keys are the names of the keyword arguments and the values are the values:
{'random': 2765, 'thing': 'gega', 'my_name': 'monkey'}
The pass
statement
Sometimes you just want an empty function. However, Python won't let you do that and putting in a comment won't work either. So, we use pass
. Simply put pass
as the code in your function and Python will do nothing:
def emptyFunction():
pass
emptyFunction()
Running a Function
Running a function quite similar to making a function, but we don't use def
and we don't type anything after the parentheses. For example, we could call the helloWorld
fucntion we made earlier by typing helloWorld()
.