Discord.py Bot Object
The Problem
If we wanted to make a real Discord bot with commands and a prefix, we could do lots and lots and lots of elif
statements in the message listener, but that would be pretty inefficient and quite ugly. Instead, we could've used Discord.py's Bot
object and set a prefix and make commands much more easily.
Using the Bot
object
The Bot
object is actually not in the main Discord.py package, but in a very useful extension subpackage called commands
which we can import by doing:
from discord.ext import commands
To create a bot object we simply write:
bot = commands.Bot(command_prefix="$")
And to run the bot:
bot.run("token")
Adding a Command
To add a command to the bot, we now use the @bot.command()
decorator for each of the bot command functions. Also, now our command functions need to have the argument ctx
as the first argument. The context is basically the context in which the message was sent (channel, author, etc.) For example:
@bot.command()
async def hello(ctx):
await ctx.send("Hello!")
Adding an Event
Events use the @bot.event()
decorator. For example, the following code prints out every message sent in the server:
@bot.event
async def on_message(message):
print(message.content)
Command Arguments
Our bot commands can also have arguments other than the context. For example:
@bot.command()
async def echo(ctx, text):
await ctx.send(text)
Here, the bot simply echoes back whatever was sent into the echo command. So, if you send $echo Hello!
, the bot sends back Hello!.
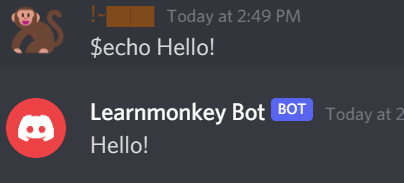