Python Counter App
In this tutorial, we will be using the Python Tkinter module to create a simple app that counts how many times the user has clicked a button.
The Tkinter Module
In this tutorial, we'll be using the Tkinter module for making graphical user interfaces (GUIs). In the last project, where we made a number guessing game, we put our game in the command line, so we made a command line interface (CLIs).
Tkinter is a built in Python module for creating GUIs. Although the apps that Tkinter makes aren't the prettiest, they are a good starting point and is very beginner-friendly.
If you want to learn more about the Tkinter module and all of its features, check out the Tkinter tutorial series on Learnmonkey.
Tkinter is built-in, so we don't need to install anything.
Writing the Code
Below is our code:
import tkinter as tk
window = tk.Tk()
counter = tk.Label(text="0")
counter.pack()
def increment_counter():
counter['text'] = int(counter['text']) + 1
button = tk.Button(text="Click Me!", command=increment_counter)
button.pack()
window.mainloop()
When you run it, you should see something like:
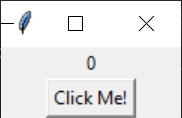
If you click the button, you should see the count above the button increase by one.
You can also find the code here.
Code Breakdown
First of all, we need to import the Tkinter module. We import it as if its name was tk
so it saves us some typing.
Then, we define a window object which you can think of as the main window of our app. We will add all of our other widgets in this window object.
We then define a Tkinter Label
object with the name of counter
, which will represent the count. Label
objects simply define some text to be shown.
After creating the Label
object, we will then have to use the pack()
method to actually put the counter in the window.
Then, we create a function called increment_counter
which increments the counter by one. We will call this function when we click on the button.
We then create a Button
object with the text of "Click Me!". We set the command
object to the increment_counter
function we defined earlier. Then, we use the pack()
method.
Finally, we run window.mainloop()
to actually display the window and listen for events like button clicks.
Extensions
Fun extension ideas:
- Make the GUI look prettier
- Make multiple buttons